Top 39 Python Interview Question For 2024
.What is Python Data Structure?What is the slots attribute in Python?What are Python's dataclasses and how are they used?.How does Python’s multiprocessing module differ from threading?
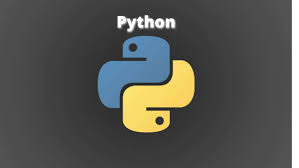
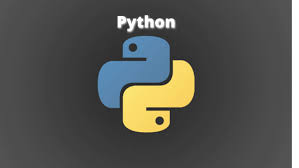
Python, created by Guido van Rossum and first introduced on February 20, 1991, is among the most widely used programming languages today. Its flexibility and dynamic semantics, combined with its open-source nature and clean, simple syntax, make Python a preferred choice for developers. This ease of learning and understanding is enhanced by Python’s support for object-oriented programming and general-purpose programming.
Basic Level Interview Questions:
1.What is Python ?
Python is a high-level, interpreted programming language known for its readability, simplicity, and versatility. Created by Guido van Rossum and first released in 1991, Python has grown to become one of the most popular programming languages in the world. Here are some key characteristics and uses of Python:
Key Characteristics:
Readability and Simplicity: Python's syntax is designed to be easy to read and write, making it an excellent choice for beginners as well as experienced developers.
Interpreted Language: Python is an interpreted language, which means that code is executed line by line, allowing for quick debugging and interactive programming.
Dynamically Typed: Variables in Python do not require explicit declaration of their type, which simplifies coding but requires careful handling of variable types.
Object-Oriented: Python supports object-oriented programming (OOP) principles, such as classes and inheritance, which help organize and structure code.
Extensive Standard Library: Python comes with a comprehensive standard library that includes modules and functions for various tasks, from file I/O to web development and data manipulation.
Cross-Platform: Python is available on multiple platforms, including Windows, macOS, and Linux, making it highly portable.
Common Uses:
Web Development: Python frameworks like Django and Flask are widely used for developing web applications.
Data Science and Machine Learning: Libraries such as Pandas, NumPy, SciPy, and scikit-learn, along with frameworks like TensorFlow and PyTorch, make Python a popular choice in these fields.
Automation and Scripting: Python is often used for automating repetitive tasks, scripting, and process automation.
Software Development: Python is used in building various types of software applications, including desktop and mobile applications.
Scientific Computing: Researchers and scientists use Python for its powerful libraries and tools that facilitate complex scientific computations and simulations.
Networking: Python is utilized in network programming for tasks such as writing network servers and clients, and automating network operations.
2.What are the key features of Python?
Python has several key features that contribute to its popularity and versatility as a programming language. Here are some of the most notable ones:
Key Features:
Readability and Simple Syntax:
Python's syntax is designed to be readable and straightforward, making it easier for developers to write clear and concise code.
It uses indentation to define code blocks, which enhances readability.
Interpreted Language:
Python is an interpreted language, meaning that it is executed line by line. This allows for immediate feedback during development and simplifies debugging.
Dynamically Typed:
Variables in Python do not require explicit type declarations. The type is determined at runtime, which simplifies code writing but requires careful handling to avoid type errors.
High-Level Language:
Python abstracts many of the complex details of the computer, such as memory management, allowing developers to focus more on programming logic rather than hardware intricacies.
Object-Oriented Programming (OOP):
Python supports object-oriented programming, allowing developers to create reusable code through classes and objects.
It also supports other programming paradigms, such as procedural and functional programming.
Extensive Standard Library:
Python comes with a comprehensive standard library that provides modules and functions for a wide range of tasks, including file I/O, system calls, web development, and more.
This extensive library reduces the need to write code from scratch for common tasks.
Cross-Platform Compatibility:
Python is available on multiple platforms, including Windows, macOS, Linux, and more. Python programs can run on any of these platforms without requiring significant changes to the code.
Integrated Development Environments (IDEs):
Python is supported by several powerful IDEs, such as PyCharm, Visual Studio Code, and Jupyter Notebook, which provide features like code completion, debugging, and interactive testing.
Large Community and Ecosystem:
Python has a vast and active community that contributes to a rich ecosystem of libraries, frameworks, and tools.
This community support makes it easier to find resources, tutorials, and third-party modules to extend Python's functionality.
Robust Frameworks and Libraries:
Python boasts a wide array of frameworks and libraries for various applications, including Django and Flask for web development, Pandas and NumPy for data analysis, TensorFlow and PyTorch for machine learning, and more.
Automation and Scripting:
Python is excellent for scripting and automating repetitive tasks, making it a popular choice for system administrators and developers who need to streamline workflows.
Interpreters and Interactive Shell:
Python offers an interactive shell that allows developers to test snippets of code quickly, facilitating a more interactive and explorative coding process.
The IDLE (Integrated Development and Learning Environment) comes with Python and provides a basic but useful environment for beginners.
Extensibility and Embeddability:
Python can be extended with C or C++ code, and it can be embedded within applications to provide scripting capabilities.
Memory Management:
Python has built-in garbage collection, which automatically handles memory management and allocation, reducing the risk of memory leaks.
3.What is Python Data Structure?
In Python, data structures are fundamental constructs that allow you to store, organize, and manage data efficiently. Python provides several built-in data structures, each suited for different types of operations and use cases. Here's an overview of the primary Python data structures:
1.List
Characteristics:
Ordered: Elements have a specific order.
Mutable: Elements can be added, removed, or changed.
Dynamic: Lists can grow and shrink in size dynamically.
Operations:
Indexing: Access elements by their position.
Slicing: Retrieve a subset of elements.
Appending: Add elements to the end.
Inserting: Insert elements at specific positions.
Deleting: Remove elements by value or position.
Iterating: Loop through elements.
Example:
# Creating a list
my_list = [1, 2, 3, 4]
# Accessing elements
print(my_list[0]) # Output: 1
# Slicing
print(my_list[1:3]) # Output: [2, 3]
# Adding elements
my_list.append(5) # my_list is now [1, 2, 3, 4, 5]
# Inserting elements
my_list.insert(2, 6) # my_list is now [1, 2, 6, 3, 4, 5]
# Removing elements
my_list.remove(6) # my_list is now [1, 2, 3, 4, 5]
print(my_list.pop(1)) # Output: 2, my_list is now [1, 3, 4, 5]
# Iterating
for item in my_list:
print(item)
Use Cases:
Dynamic arrays.
Stacks and queues (with appropriate methods).
2. Tuples
Characteristics:
Ordered: Elements have a specific order.
Immutable: Elements cannot be changed after creation.
Fixed Size: Cannot be resized after creation.
Operations:
Indexing: Access elements by their position.
Slicing: Retrieve a subset of elements.
Iterating: Loop through elements.
Examples:
# Creating a tuple
my_tuple = (1, 2, 3, 4)
# Accessing elements
print(my_tuple[0]) # Output: 1
# Slicing
print(my_tuple[1:3]) # Output: (2, 3)
# Unpacking
a, b, c, d = my_tuple
print(a, b, c, d) # Output: 1 2 3 4
# Iterating
for item in my_tuple:
print(item)
Use Cases:
Fixed collections of related items.
Returning multiple values from functions.
3. Dictionaries
Characteristics:
Unordered: No specific order.
Mutable: Key-value pairs can be added, removed, or changed.
Key-Value Pairs: Each key is unique and maps to a value.
Operations:
Accessing: Retrieve values by key.
Adding/Updating: Insert or modify key-value pairs.
Deleting: Remove key-value pairs.
Iterating: Loop through keys, values, or items.
Examples:
# Creating a dictionary
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Accessing values
print(my_dict['key1']) # Output: value1
# Adding/Updating values
my_dict['key3'] = 'value3' # my_dict is now {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
# Deleting values
del my_dict['key2'] # my_dict is now {'key1': 'value1', 'key3': 'value3'}
# Iterating
for key, value in my_dict.items():
print(key, value)
Use Cases:
Lookups and retrieval by key.
Configuration settings.
JSON-like data structures.
4. Sets
Characteristics:
Unordered: No specific order.
Mutable: Elements can be added or removed.
Unique Elements: No duplicates allowed.
Operations:
Adding: Add elements.
Removing: Remove elements.
Set Operations: Union, intersection, difference.
Membership Testing: Check if an element is in the set.
# Creating a set
my_set = {1, 2, 3, 4}
# Adding elements
my_set.add(5) # my_set is now {1, 2, 3, 4, 5}
# Removing elements
my_set.remove(3) # my_set is now {1, 2, 4, 5}
# Set operations
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1 | set2 # {1, 2, 3, 4, 5}
intersection_set = set1 & set2 # {3}
difference_set = set1 - set2 # {1, 2}
# Membership testing
print(3 in my_set) # Output: True
print(6 in my_set) # Output: False
Use Cases:
Removing duplicates from a list.
Membership testing.
Mathematical set operations.
Choosing the Right Data Structure:
List: Use when you need an ordered collection that allows duplicates and requires frequent modifications.
Tuple: Use when you need an ordered collection of fixed size that should not be modified.
Dictionary: Use when you need a collection of key-value pairs for fast lookups and updates.
Set: Use when you need a collection of unique elements and require efficient membership testing or set operations.
These data structures form the foundation of data manipulation and organization in Python, enabling efficient and effective coding for a variety of applications.
4.Difference Between List, Tuple, Sets and Dictionaries?
Lists
Ordered and Mutable.
Supports indexing and slicing.
Allows duplicates.
Use case: General-purpose collection of items where order matters.
Tuples
Ordered and Immutable.
Supports indexing.
Allows duplicates.
Use case: Fixed collections of items, useful for fixed data structures (e.g., coordinates, database records).
Sets
Unordered and Mutable (though elements must be immutable).
No indexing.
Unique elements only.
Use case: Collection of unique items, membership tests, and eliminating duplicates.
Dictionaries
Ordered and Mutable.
Indexed by unique keys (key-value pairs).
No duplicate keys (values can be duplicated).
Use case: Fast lookups, mappings, and associative arrays.
5.What is PEP 8?
PEP 8 is the style guide for Python code. It stands for Python Enhancement Proposal 8 and provides conventions for writing readable and consistent Python code. Here are some of the key aspects it covers:
Indentation:
Use 4 spaces per indentation level.
Never mix tabs and spaces.
Line Length:
Limit all lines to a maximum of 79 characters.
Blank Lines:
Surround top-level function and class definitions with two blank lines.
Method definitions inside a class are surrounded by a single blank line.
Imports:
Imports should usually be on separate lines.
Imports should be grouped in the following order: standard library imports, related third-party imports, local application/library-specific imports.
Whitespace in Expressions and Statements:
Avoid extraneous whitespace in the following situations:
Immediately inside parentheses, brackets, or braces.
Between a trailing comma and a following close parenthesis.
Immediately before a comma, semicolon, or colon.
Immediately before the open parenthesis that starts the argument list of a function call.
Immediately before the open parenthesis that starts an indexing or slicing.
Use a single space around operators and after commas, colons, and semicolons.
Comments:
Use comments to explain code.
Use docstrings for all public modules, functions, classes, and methods.
Comments should be complete sentences and should start with a capital letter.
Naming Conventions:
Use CapWords for class names.
Use lowercase_with_underscores for function and variable names.
Constants should be UPPERCASE_WITH_UNDERSCORES.
Programming Recommendations:
Avoid using global variables.
Use is or is not when comparing to None.
PEP 8 aims to improve the readability of Python code and make it consistent across the Python community, which helps developers understand and collaborate on code more easily.
6.What do you mean by Python literals?
In Python, literals are data values that are fixed and directly written into your code. They represent raw data that can't be changed. Python supports various types of literals:
Numeric literals: These include integers, floating-point numbers, and complex numbers. For example:
Integers: 42, -10, 0
Floating-point numbers: 3.14, 2.71828
Complex numbers: 3 + 4j, 1 - 2j
String literals: These represent textual data. They can be enclosed in single quotes ('), double quotes ("), or triple quotes (''' or """). For example:
Single-line strings: 'Hello', "World"
Multi-line strings: '''Lorem ipsum dolor sit amet, consectetur adipiscing elit.'''
Boolean literals: These represent truth values. They can be either True or False.
None literal: This represents the absence of a value. It's denoted by the keyword None.
Sequence literals:
List literals: [1, 2, 3]
Tuple literals: (1, 2, 3)
Set literals: {1, 2, 3}
Dictionary literals: {'a': 1, 'b': 2}
Special literals: These include True, False, and None.
Python literals are used to initialize variables or provide data directly in your code.
7.What is the Global Interpreter Lock (GIL)?
The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes simultaneously in the same process. This can limit the performance of CPU-bound and multithreaded programs but helps in single-threaded performance and integration with C libraries
8.What are decorators in Python?
Decorators are a way to modify the behavior of a function or method. They are defined using the decorator_name syntax above the function definition and can be used to add functionality to existing code in a clean and reusable way.
9.Explain the difference between shallow and deep copying.
Shallow copying creates a new object but inserts references into it to the objects found in the original. Deep copying creates a new object and recursively copies all objects found in the original, leading to entirely independent objects.
10.What are Python’s generators and the yield keyword?
Generators are a type of iterator that allows you to iterate over a sequence of values without storing them all in memory. They are defined using functions with the yield keyword, which returns values one at a time and remembers the state of the function between calls.
11.What are context managers and the with statement?
Context managers allow for resource management (like file operations) and are implemented using the enter and exit methods. The with statement ensures that resources are properly acquired and released, even if an error occurs during the block execution.
12.What is the difference between str and repr in Python?
The str method provides a readable, user-friendly representation of an object, while repr provides an unambiguous representation, useful for developers. If str is not defined, repr is used as a fallback.
13.Explain the concept of metaclasses in Python?
Metaclasses are the 'classes of classes' in Python. They define how classes behave. By default, Python uses type as the metaclass, but you can create custom metaclasses by inheriting from type and overriding its methods. Metaclasses are powerful tools for modifying class creation and behavior.
14.What are descriptors and how are they used in Python?
Descriptors are objects that define how attributes are accessed, modified, and deleted in a class. They implement one or more of the special methods get, set, and delete. Descriptors are often used to create properties, methods, and class methods.
15.How does Python's garbage collection work?
Python uses a combination of reference counting and a cycle-detecting garbage collector to manage memory. Reference counting keeps track of the number of references to each object. When the reference count drops to zero, the object is deallocated. The garbage collector detects and cleans up reference cycles that reference counting alone cannot handle.
16.What are context managers and how are they implemented in Python?
Context managers allow you to allocate and release resources precisely when you want to. The with statement is used with context managers to ensure that resources are properly managed. They are implemented using the enter and exit methods.
17.What is the difference between str and repr methods?
The str method is used to provide a readable, user-friendly representation of an object, while repr is used to provide an unambiguous representation, primarily for debugging and development. If str is not defined, repr is used as a fallback.
18.Explain the concept of metaclasses with an example.
Metaclasses can be used to automatically register classes, enforce coding standards, or modify class creation. Example:
class MyMeta(type):
def new(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
19.What is duck typing in Python?
Duck typing is a concept where the type or class of an object is less important than the methods it defines or the behavior it exhibits. If an object behaves like a duck (has a quack method), it can be treated as a duck.
20.What are Python’s property decorator and its uses?
The property decorator allows you to define methods in a class that can be accessed like attributes. It is used to encapsulate instance variables and provide a controlled way to get, set, and delete their values.
21.What is a closure in Python?
A closure is a function object that retains access to variables from its containing (enclosing) lexical scope, even after the scope has finished execution. Closures are created when a nested function references variables from its enclosing function
22.How do you handle exceptions in Python?
Python uses try, except, else, and finally blocks to handle exceptions. try block contains the code that might raise an exception, except handles the exception, else runs if no exception occurs, and finally runs irrespective of whether an exception occurred or not.
23.What are Python’s slots and how do they optimize memory usage?
slots is a special attribute that restricts the attributes that can be added to an instance of a class, thus saving memory by preventing the creation of dict for each instance.
24.What is the purpose of the nonlocal keyword in Python?
The nonlocal keyword allows you to assign values to variables in an enclosing (but non-global) scope. It is used within nested functions to modify variables in the outer function.
25.What is the difference between the is and == operators?
is: Checks for object identity, meaning it returns True if two references point to the same object.
==: Checks for value equality, meaning it returns True if two objects have the same value.
26.What are Python’s abstract base classes (ABCs) and why are they used?
ABCs, defined in the abc module, provide a way to define abstract methods that must be implemented by derived classes. They help enforce certain interfaces and provide a blueprint for subclasses.
27.How does Python’s asyncio module work?
The asyncio module provides support for asynchronous I/O, event loops, coroutines, and tasks. It enablesHere are the remaining advanced-level Python interview questions with detailed explanations:
28.How do you implement method chaining in Python?
Method chaining is achieved by returning self from methods so that multiple methods can be called on the same object in a single line.
class MyClass:
def method1(self):
print("Method 1")
return self
def method2(self):
print("Method 2")
return self
obj = MyClass()
obj.method1().method2()
29.What are descriptors and how are they used?
Descriptors are objects that manage the access to another object's attributes. They define methods get, set, and delete.
class Descriptor:
def get(self, instance, owner):
return instance.__dict__.get(self.name)
def set(self, instance, value):
instance.__dict__[self.name] = value
class MyClass:
attribute = Descriptor()
30.How does the with statement work in Python?
The with statement simplifies exception handling by encapsulating common preparation and cleanup tasks. It makes use of context managers defined by enter and exit methods.
class MyContext:
def enter(self):
print("Entering context")
return self
def exit(self, exc_type, exc_val, exc_tb):
print("Exiting context")
with MyContext():
print("Inside context")
31.Explain the use of the super() function.
The super() function returns a temporary object of the superclass, allowing you to call its methods. It is commonly used to call the parent class's methods from within a child class.
class Parent:
def init(self):
print("Parent init")
class Child(Parent):
def init(self):
super().__init__()
print("Child init")
32.What are function annotations and how are they used?
Function annotations are a way of associating arbitrary metadata with function arguments and return values. They are defined using a colon (:) after the parameter name and before the equal sign.
def func(x: int, y: int) -> int:
return x + y
33.What is the slots attribute in Python?
The slots attribute is used to restrict the attributes that instances of a class can have, which can save memory by preventing the creation of dict for each instance.
class MyClass:
slots = ['attribute1', 'attribute2']
34.What are Python's dataclasses and how are they used?
Introduced in Python 3.7, dataclasses provide a decorator and functions for automatically adding special methods to user-defined classes. They simplify the creation of classes that primarily store data by generating methods like init, repr, and eq.
35.How does method resolution order (MRO) work in Python?
Python uses the C3 linearization algorithm to determine the method resolution order. MRO defines the order in which base classes are searched when executing a method. It is crucial in multiple inheritance to avoid conflicts and ensure consistent behavior.
36.What is a coroutine in Python and how is it different from a generator?
Coroutines are generalized forms of generators. While generators produce values, coroutines can consume and produce values, and they support asynchronous programming with async and await syntax introduced in Python.
37.Explain the difference between staticmethod, classmethod, and instance methods.
Instance methods: Operate on an instance of the class and can access and modify the instance attributes. They are defined with def method(self).
Class methods: Operate on the class itself and can modify class state. They are defined with def method(cls) and use the @classmethod decorator.
Static methods: Do not operate on an instance or class and do not access or modify state. They are defined with def method() and use the @staticmethod decorator.
38.How does Python’s multiprocessing module differ from threading?
The multiprocessing module allows you to create processes that run in separate memory spaces, avoiding the GIL's limitations. The threading module creates threads within the same process, sharing memory space but restricted by the GIL.
39.How are Python’s functools and itertools modules used?
functools: Provides higher-order functions that operate on other functions, such as partial, reduce, and lru_cache.
itertools: Provides functions for creating iterators for efficient looping, such as chain, cycle, and combinations.